What Is The Eax Register
x86 Associates Guide
Contents: Registers | Memory and Addressing | Instructions | Calling Convention
This guide describes the nuts of 32-fleck x86 assembly language programming, covering a small but useful subset of the available instructions and assembler directives. In that location are several different assembly languages for generating x86 machine code. The one we volition use in CS216 is the Microsoft Macro Assembler (MASM) assembler. MASM uses the standard Intel syntax for writing x86 assembly lawmaking.
The full x86 pedagogy gear up is large and complex (Intel'southward x86 instruction set manuals incorporate over 2900 pages), and we practise non cover information technology all in this guide. For example, there is a xvi-bit subset of the x86 pedagogy fix. Using the 16-bit programming model tin be quite complex. It has a segmented memory model, more restrictions on annals usage, and and then on. In this guide, we will limit our attention to more modernistic aspects of x86 programming, and delve into the educational activity gear up only in enough item to get a basic feel for x86 programming.
Resource
- Guide to Using Associates in Visual Studio — a tutorial on building and debugging assembly lawmaking in Visual Studio
- Intel x86 Pedagogy Ready Reference
- Intel's Pentium Manuals (the full gory details)
Registers
Modern (i.e 386 and beyond) x86 processors have eight 32-bit full general purpose registers, as depicted in Figure 1. The register names are mostly historical. For example, EAX used to be chosen the accumulator since it was used by a number of arithmetics operations, and ECX was known every bit the counter since it was used to concord a loop index. Whereas most of the registers have lost their special purposes in the modern teaching set, past convention, two are reserved for special purposes — the stack arrow (ESP) and the base pointer (EBP).
For the EAX, EBX, ECX, and EDX registers, subsections may be used. For case, the least pregnant 2 bytes of EAX can be treated as a 16-scrap register called AX. The least meaning byte of AX tin exist used as a single eight-bit register chosen AL, while the near significant byte of AX can be used as a single 8-flake annals chosen AH. These names refer to the aforementioned physical annals. When a two-byte quantity is placed into DX, the update affects the value of DH, DL, and EDX. These sub-registers are mainly hold-overs from older, 16-scrap versions of the instruction set. All the same, they are sometimes convenient when dealing with data that are smaller than 32-bits (e.g. 1-byte ASCII characters).
When referring to registers in assembly language, the names are not example-sensitive. For example, the names EAX and eax refer to the aforementioned annals.
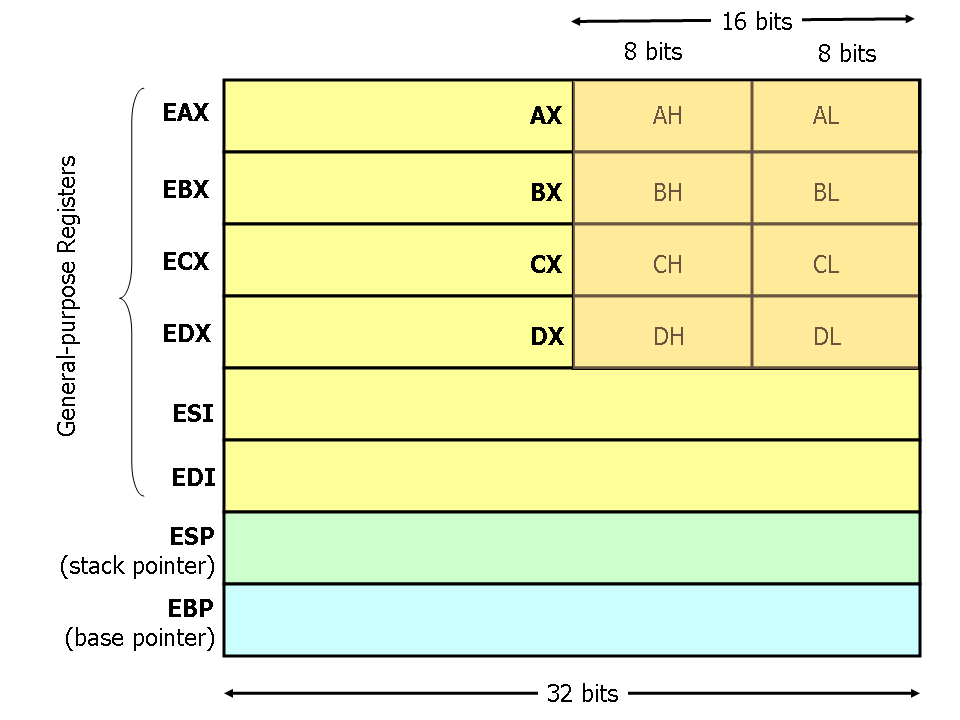
Figure 1. x86 Registers
Retentiveness and Addressing Modes
Declaring Static Data Regions
You tin can declare static data regions (analogous to global variables) in x86 associates using special assembler directives for this purpose. Information declarations should exist preceded by the .DATA directive. Following this directive, the directives DB, DW, and DD tin can be used to declare one, ii, and 4 byte data locations, respectively. Declared locations can be labeled with names for later reference — this is similar to declaring variables by proper name, but abides by some lower level rules. For example, locations declared in sequence will be located in memory side by side to one another.
Instance declarations:
.Information var DB 64 ; Declare a byte, referred to equally location var, containing the value 64. var2 DB ? ; Declare an uninitialized byte, referred to equally location var2. DB ten ; Declare a byte with no label, containing the value 10. Its location is var2 + 1. X DW ? ; Declare a 2-byte uninitialized value, referred to as location 10. Y DD 30000 ; Declare a 4-byte value, referred to as location Y, initialized to 30000.
Unlike in high level languages where arrays tin have many dimensions and are accessed by indices, arrays in x86 assembly language are simply a number of cells located contiguously in memory. An array tin can be declared by just listing the values, as in the first example beneath. Ii other common methods used for declaring arrays of data are the DUP directive and the use of string literals. The DUP directive tells the assembler to indistinguishable an expression a given number of times. For example, iv DUP(2) is equivalent to 2, 2, two, 2.
Some examples:
Z DD i, 2, iii ; Declare three 4-byte values, initialized to one, 2, and 3. The value of location Z + 8 will exist 3. bytes DB 10 DUP(?) ; Declare 10 uninitialized bytes starting at location bytes. arr DD 100 DUP(0) ; Declare 100 4-byte words starting at location arr, all initialized to 0 str DB 'hello',0 ; Declare 6 bytes starting at the address str, initialized to the ASCII character values for hello and the null (0) byte.
Addressing Memory
Modernistic x86-compatible processors are capable of addressing upward to 232 bytes of memory: memory addresses are 32-bits wide. In the examples higher up, where we used labels to refer to retention regions, these labels are actually replaced by the assembler with 32-bit quantities that specify addresses in memory. In add-on to supporting referring to memory regions by labels (i.e. constant values), the x86 provides a flexible scheme for computing and referring to memory addresses: upward to ii of the 32-chip registers and a 32-scrap signed constant tin be added together to compute a memory address. One of the registers can be optionally pre-multiplied past 2, 4, or 8.
The addressing modes can exist used with many x86 instructions (we'll describe them in the next section). Here we illustrate some examples using the mov didactics that moves data between registers and retentiveness. This instruction has two operands: the beginning is the destination and the second specifies the source.
Some examples of mov instructions using address computations are:
mov eax, [ebx] ; Movement the 4 bytes in memory at the address contained in EBX into EAX mov [var], ebx ; Move the contents of EBX into the 4 bytes at memory address var. (Note, var is a 32-bit constant). mov eax, [esi-4] ; Move 4 bytes at retentivity accost ESI + (-four) into EAX mov [esi+eax], cl ; Motility the contents of CL into the byte at accost ESI+EAX mov edx, [esi+4*ebx] ; Move the 4 bytes of data at address ESI+4*EBX into EDX
Some examples of invalid address calculations include:
mov eax, [ebx-ecx] ; Tin can but add register values mov [eax+esi+edi], ebx ; At most 2 registers in accost computation
Size Directives
In general, the intended size of the data item at a given memory address tin can be inferred from the assembly code instruction in which it is referenced. For instance, in all of the above instructions, the size of the memory regions could exist inferred from the size of the register operand. When nosotros were loading a 32-bit register, the assembler could infer that the region of memory we were referring to was 4 bytes broad. When we were storing the value of a one byte annals to memory, the assembler could infer that we wanted the address to refer to a single byte in memory.
However, in some cases the size of a referred-to memory region is ambiguous. Consider the instruction mov [ebx], 2. Should this instruction motility the value 2 into the single byte at accost EBX? Perhaps it should move the 32-chip integer representation of ii into the 4-bytes starting at accost EBX. Since either is a valid possible interpretation, the assembler must exist explicitly directed equally to which is correct. The size directives BYTE PTR, Word PTR, and DWORD PTR serve this purpose, indicating sizes of ane, 2, and four bytes respectively.
For example:
mov BYTE PTR [ebx], two ; Move two into the unmarried byte at the address stored in EBX. mov WORD PTR [ebx], 2 ; Move the 16-bit integer representation of 2 into the 2 bytes starting at the address in EBX. mov DWORD PTR [ebx], ii ; Movement the 32-scrap integer representation of 2 into the 4 bytes starting at the accost in EBX.
Instructions
Auto instructions generally fall into three categories: data motion, arithmetic/logic, and command-flow. In this department, we will look at important examples of x86 instructions from each category. This section should not be considered an exhaustive list of x86 instructions, but rather a useful subset. For a consummate list, see Intel'due south instruction set reference.
Nosotros use the following notation:
<reg32> Any 32-bit register (EAX, EBX, ECX, EDX, ESI, EDI, ESP, or EBP) <reg16> Any 16-bit annals (AX, BX, CX, or DX) <reg8> Any 8-bit register (AH, BH, CH, DH, AL, BL, CL, or DL) <reg> Whatsoever annals <mem> A memory address (e.g., [eax], [var + iv], or dword ptr [eax+ebx]) <con32> Any 32-bit abiding <con16> Any 16-flake abiding <con8> Any eight-bit constant <con> Any 8-, 16-, or 32-scrap constant
Data Movement Instructions
mov — Move (Opcodes: 88, 89, 8A, 8B, 8C, 8E, ...)
The mov instruction copies the data particular referred to by its second operand (i.e. register contents, memory contents, or a constant value) into the location referred to by its showtime operand (i.eastward. a annals or retentivity). While register-to-register moves are possible, direct memory-to-retentiveness moves are not. In cases where memory transfers are desired, the source retentiveness contents must first exist loaded into a register, and then can exist stored to the destination retention address.Syntax
mov <reg>,<reg>
mov <reg>,<mem>
mov <mem>,<reg>
mov <reg>,<const>
mov <mem>,<const>
Examples
mov eax, ebx — copy the value in ebx into eax
mov byte ptr [var], five — store the value 5 into the byte at location var
push — Button stack (Opcodes: FF, 89, 8A, 8B, 8C, 8E, ...)
The push instruction places its operand onto the tiptop of the hardware supported stack in retentivity. Specifically, push kickoff decrements ESP by 4, so places its operand into the contents of the 32-bit location at address [ESP]. ESP (the stack pointer) is decremented by push since the x86 stack grows down - i.e. the stack grows from high addresses to lower addresses. Syntax
push button <reg32>
button <mem>
push <con32>Examples
push eax — push eax on the stack
push [var] — push the 4 bytes at address var onto the stack
pop — Pop stack
The pop instruction removes the four-byte data element from the top of the hardware-supported stack into the specified operand (i.eastward. register or memory location). Information technology first moves the 4 bytes located at memory location [SP] into the specified register or memory location, and then increments SP past iv.Syntax
Examples
pop <reg32>
pop <mem>
pop edi — pop the top element of the stack into EDI.
pop [ebx] — pop the top element of the stack into retentivity at the 4 bytes starting at location EBX.
lea — Load effective address
The lea teaching places the address specified by its second operand into the register specified by its first operand. Notation, the contents of the retention location are not loaded, only the effective accost is computed and placed into the annals. This is useful for obtaining a pointer into a memory region.Syntax
lea <reg32>,<mem>Examples
lea edi, [ebx+4*esi] — the quantity EBX+4*ESI is placed in EDI.
lea eax, [var] — the value in var is placed in EAX.
lea eax, [val] — the value val is placed in EAX.
Arithmetic and Logic Instructions
add — Integer Improver
The add instruction adds together its ii operands, storing the result in its offset operand. Notation, whereas both operands may be registers, at most i operand may be a retentiveness location. Syntax
add together <reg>,<reg>
add <reg>,<mem>
add together <mem>,<reg>
add together <reg>,<con>
add <mem>,<con>
Examples
add eax, ten — EAX ← EAX + 10
add BYTE PTR [var], x — add 10 to the single byte stored at memory address var
sub — Integer Subtraction
The sub instruction stores in the value of its first operand the consequence of subtracting the value of its second operand from the value of its first operand. As with add together Syntax
sub <reg>,<reg>
sub <reg>,<mem>
sub <mem>,<reg>
sub <reg>,<con>
sub <mem>,<con>
Examples
sub al, ah — AL ← AL - AH
sub eax, 216 — subtract 216 from the value stored in EAX
inc, dec — Increment, Decrement
The inc educational activity increments the contents of its operand by one. The dec instruction decrements the contents of its operand past one.Syntax
inc <reg>
inc <mem>
dec <reg>
dec <mem>Examples
dec eax — subtract ane from the contents of EAX.
inc DWORD PTR [var] — add one to the 32-fleck integer stored at location var
imul — Integer Multiplication
The imul instruction has two bones formats: 2-operand (first two syntax listings above) and 3-operand (last two syntax listings higher up). The ii-operand form multiplies its two operands together and stores the consequence in the first operand. The consequence (i.east. outset) operand must be a register. The three operand course multiplies its second and tertiary operands together and stores the effect in its first operand. Once again, the outcome operand must be a register. Furthermore, the third operand is restricted to being a constant value. Syntax
imul <reg32>,<reg32>
imul <reg32>,<mem>
imul <reg32>,<reg32>,<con>
imul <reg32>,<mem>,<con>Examples
imul eax, [var] — multiply the contents of EAX by the 32-bit contents of the memory location var. Store the outcome in EAX.
imul esi, edi, 25 — ESI → EDI * 25
idiv — Integer Sectionalisation
The idiv education divides the contents of the 64 bit integer EDX:EAX (constructed by viewing EDX every bit the most pregnant 4 bytes and EAX as the to the lowest degree meaning four bytes) past the specified operand value. The quotient result of the division is stored into EAX, while the balance is placed in EDX.Syntax
idiv <reg32>
idiv <mem>Examples
idiv ebx — split the contents of EDX:EAX past the contents of EBX. Place the caliber in EAX and the remainder in EDX.
idiv DWORD PTR [var] — divide the contents of EDX:EAX by the 32-chip value stored at memory location var. Place the caliber in EAX and the remainder in EDX.
and, or, xor — Bitwise logical and, or and sectional or
These instructions perform the specified logical operation (logical bitwise and, or, and exclusive or, respectively) on their operands, placing the result in the first operand location.Syntax
and <reg>,<reg>
and <reg>,<mem>
and <mem>,<reg>
and <reg>,<con>
and <mem>,<con>
or <reg>,<reg>
or <reg>,<mem>
or <mem>,<reg>
or <reg>,<con>
or <mem>,<con>
xor <reg>,<reg>
xor <reg>,<mem>
xor <mem>,<reg>
xor <reg>,<con>
xor <mem>,<con>
Examples
and eax, 0fH — articulate all just the terminal four $.25 of EAX.
xor edx, edx — set the contents of EDX to zero.
not — Bitwise Logical Not
Logically negates the operand contents (that is, flips all scrap values in the operand).Syntax
non <reg>
non <mem>Instance
not BYTE PTR [var] — negate all bits in the byte at the memory location var.
neg — Negate
Performs the 2'south complement negation of the operand contents.Syntax
neg <reg>
neg <mem>Example
neg eax — EAX → - EAX
shl, shr — Shift Left, Shift Right
These instructions shift the bits in their first operand'south contents left and right, padding the resulting empty chip positions with zeros. The shifted operand tin can be shifted upwardly to 31 places. The number of bits to shift is specified by the 2nd operand, which tin be either an 8-fleck constant or the register CL. In either instance, shifts counts of greater then 31 are performed modulo 32.Syntax
shl <reg>,<con8>
shl <mem>,<con8>
shl <reg>,<cl>
shl <mem>,<cl>shr <reg>,<con8>
shr <mem>,<con8>
shr <reg>,<cl>
shr <mem>,<cl>Examples
shl eax, 1 — Multiply the value of EAX by 2 (if the most significant chip is 0)
shr ebx, cl — Store in EBX the floor of effect of dividing the value of EBX by 2 north wheren is the value in CL.
Control Flow Instructions
The x86 processor maintains an educational activity arrow (IP) annals that is a 32-bit value indicating the location in retentivity where the current instruction starts. Usually, it increments to point to the next instruction in memory begins after execution an educational activity. The IP register cannot be manipulated directly, merely is updated implicitly past provided control flow instructions.
We utilize the notation <label> to refer to labeled locations in the program text. Labels can be inserted anywhere in x86 assembly code text by entering a label name followed past a colon. For example,
mov esi, [ebp+viii] begin: xor ecx, ecx mov eax, [esi]
The second teaching in this lawmaking fragment is labeled begin. Elsewhere in the code, nosotros tin can refer to the memory location that this pedagogy is located at in memory using the more convenient symbolic proper name begin. This characterization is but a convenient manner of expressing the location instead of its 32-flake value.
jmp — Jump
Transfers program control period to the instruction at the memory location indicated by the operand.Syntax
jmp <label>Example
jmp begin — Spring to the education labeled begin.
jcondition — Provisional Spring
These instructions are conditional jumps that are based on the status of a set of condition codes that are stored in a special annals called the machine condition word. The contents of the motorcar status discussion include data well-nigh the last arithmetics operation performed. For example, one chip of this word indicates if the last result was null. Some other indicates if the last result was negative. Based on these condition codes, a number of conditional jumps can be performed. For case, the jz didactics performs a leap to the specified operand label if the result of the last arithmetic performance was nix. Otherwise, control gain to the adjacent teaching in sequence.A number of the provisional branches are given names that are intuitively based on the last operation performed being a special compare instruction, cmp (see below). For example, conditional branches such equally jle and jne are based on first performing a cmp operation on the desired operands.
Syntax
je <label> (jump when equal)
jne <characterization> (jump when not equal)
jz <label> (jump when last upshot was zero)
jg <characterization> (jump when greater than)
jge <label> (leap when greater than or equal to)
jl <label> (bound when less than)
jle <label> (jump when less than or equal to)Instance
cmp eax, ebx
jle washedIf the contents of EAX are less than or equal to the contents of EBX, jump to the label done. Otherwise, continue to the next instruction.
cmp — Compare
Compare the values of the ii specified operands, setting the status codes in the machine condition discussion appropriately. This instruction is equivalent to the sub instruction, except the upshot of the subtraction is discarded instead of replacing the start operand.Syntax
cmp <reg>,<reg>
cmp <reg>,<mem>
cmp <mem>,<reg>
cmp <reg>,<con>Example
cmp DWORD PTR [var], 10
jeq loopIf the 4 bytes stored at location var are equal to the iv-byte integer constant 10, spring to the location labeled loop.
telephone call, ret — Subroutine telephone call and return
These instructions implement a subroutine call and render. The call instruction first pushes the current code location onto the hardware supported stack in memory (see the button didactics for details), and so performs an unconditional jump to the code location indicated by the label operand. Unlike the uncomplicated jump instructions, the telephone call instruction saves the location to render to when the subroutine completes.The ret instruction implements a subroutine return machinery. This instruction start pops a code location off the hardware supported in-retentiveness stack (run into the pop instruction for details). Information technology then performs an unconditional jump to the retrieved code location.
Syntax
call <label>
ret
Calling Convention
To allow divide programmers to share code and develop libraries for use by many programs, and to simplify the use of subroutines in full general, programmers typically adopt a mutual calling convention. The calling convention is a protocol about how to call and render from routines. For example, given a fix of calling convention rules, a programmer need not examine the definition of a subroutine to determine how parameters should exist passed to that subroutine. Furthermore, given a gear up of calling convention rules, high-level language compilers can exist made to follow the rules, thus assuasive hand-coded assembly language routines and loftier-level language routines to telephone call one some other.
In practice, many calling conventions are possible. Nosotros volition utilize the widely used C linguistic communication calling convention. Post-obit this convention will let you to write associates language subroutines that are safely callable from C (and C++) lawmaking, and will also enable you to call C library functions from your assembly language code.
The C calling convention is based heavily on the utilize of the hardware-supported stack. Information technology is based on the push, popular, telephone call, and ret instructions. Subroutine parameters are passed on the stack. Registers are saved on the stack, and local variables used by subroutines are placed in retentivity on the stack. The vast majority of high-level procedural languages implemented on nearly processors have used similar calling conventions.
The calling convention is broken into two sets of rules. The first set up of rules is employed by the caller of the subroutine, and the second fix of rules is observed by the writer of the subroutine (the callee). It should be emphasized that mistakes in the observance of these rules speedily outcome in fatal program errors since the stack will be left in an inconsistent state; thus meticulous care should be used when implementing the call convention in your own subroutines.
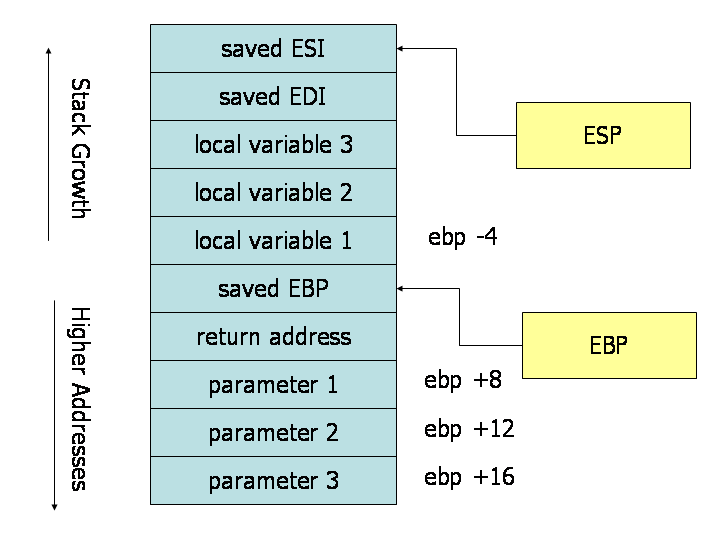
Stack during Subroutine Call
[Thanks to Maxence Faldor for providing a right figure and to James Peterson for finding and fixing the bug in the original version of this figure!]
A good way to visualize the performance of the calling convention is to draw the contents of the nearby region of the stack during subroutine execution. The image above depicts the contents of the stack during the execution of a subroutine with 3 parameters and three local variables. The cells depicted in the stack are 32-bit wide memory locations, thus the memory addresses of the cells are 4 bytes apart. The starting time parameter resides at an offset of viii bytes from the base pointer. Above the parameters on the stack (and below the base of operations arrow), the telephone call educational activity placed the return address, thus leading to an extra four bytes of outset from the base pointer to the offset parameter. When the ret instruction is used to return from the subroutine, it will jump to the render address stored on the stack.
Caller Rules
To make a subrouting call, the caller should:
- Earlier calling a subroutine, the caller should salve the contents of certain registers that are designated caller-saved. The caller-saved registers are EAX, ECX, EDX. Since the chosen subroutine is allowed to modify these registers, if the caller relies on their values after the subroutine returns, the caller must push button the values in these registers onto the stack (so they can be restore later on the subroutine returns.
- To pass parameters to the subroutine, push them onto the stack earlier the telephone call. The parameters should be pushed in inverted society (i.e. concluding parameter first). Since the stack grows down, the first parameter volition be stored at the lowest address (this inversion of parameters was historically used to allow functions to be passed a variable number of parameters).
- To phone call the subroutine, use the telephone call education. This pedagogy places the return address on top of the parameters on the stack, and branches to the subroutine lawmaking. This invokes the subroutine, which should follow the callee rules beneath.
Subsequently the subroutine returns (immediately following the call pedagogy), the caller can expect to find the return value of the subroutine in the annals EAX. To restore the machine state, the caller should:
- Remove the parameters from stack. This restores the stack to its state earlier the phone call was performed.
- Restore the contents of caller-saved registers (EAX, ECX, EDX) past popping them off of the stack. The caller can assume that no other registers were modified by the subroutine.
Example
The code beneath shows a function phone call that follows the caller rules. The caller is calling a function _myFunc that takes three integer parameters. Outset parameter is in EAX, the 2nd parameter is the constant 216; the tertiary parameter is in retention location var.
push [var] ; Push last parameter beginning push button 216 ; Push the second parameter button eax ; Push first parameter last phone call _myFunc ; Call the function (assume C naming) add together esp, 12
Note that after the call returns, the caller cleans upwardly the stack using the add instruction. We have 12 bytes (three parameters * 4 bytes each) on the stack, and the stack grows down. Thus, to get rid of the parameters, nosotros can but add 12 to the stack pointer.
The outcome produced by _myFunc is now available for use in the register EAX. The values of the caller-saved registers (ECX and EDX), may have been changed. If the caller uses them after the call, it would have needed to salvage them on the stack before the telephone call and restore them after it.
Callee Rules
The definition of the subroutine should adhere to the following rules at the start of the subroutine:
- Push the value of EBP onto the stack, and then re-create the value of ESP into EBP using the post-obit instructions:
push ebp mov ebp, esp
This initial action maintains the base of operations pointer, EBP. The base of operations pointer is used by convention as a point of reference for finding parameters and local variables on the stack. When a subroutine is executing, the base pointer holds a copy of the stack pointer value from when the subroutine started executing. Parameters and local variables volition always exist located at known, constant offsets away from the base of operations pointer value. We push the erstwhile base of operations pointer value at the get-go of the subroutine then that we can later restore the appropriate base of operations pointer value for the caller when the subroutine returns. Remember, the caller is not expecting the subroutine to change the value of the base of operations arrow. We then move the stack pointer into EBP to obtain our bespeak of reference for accessing parameters and local variables. - Next, classify local variables by making space on the stack. Remember, the stack grows down, so to make infinite on the top of the stack, the stack arrow should be decremented. The amount past which the stack pointer is decremented depends on the number and size of local variables needed. For example, if three local integers (4 bytes each) were required, the stack pointer would need to be decremented by 12 to make infinite for these local variables (i.east., sub esp, 12). As with parameters, local variables will be located at known offsets from the base of operations pointer.
- Next, save the values of the callee-saved registers that volition be used past the function. To relieve registers, push them onto the stack. The callee-saved registers are EBX, EDI, and ESI (ESP and EBP will also exist preserved by the calling convention, but demand not be pushed on the stack during this step).
Afterwards these three actions are performed, the body of the subroutine may keep. When the subroutine is returns, it must follow these steps:
- Leave the return value in EAX.
- Restore the old values of any callee-saved registers (EDI and ESI) that were modified. The register contents are restored by popping them from the stack. The registers should exist popped in the inverse order that they were pushed.
- Deallocate local variables. The obvious style to practice this might exist to add the appropriate value to the stack arrow (since the space was allocated by subtracting the needed amount from the stack pointer). In practice, a less error-prone way to deallocate the variables is to move the value in the base of operations pointer into the stack pointer: mov esp, ebp. This works because the base pointer e'er contains the value that the stack pointer independent immediately prior to the allocation of the local variables.
- Immediately before returning, restore the caller's base arrow value past popping EBP off the stack. Recall that the first matter we did on entry to the subroutine was to push button the base pointer to save its quondam value.
- Finally, return to the caller by executing a ret instruction. This instruction volition detect and remove the advisable return accost from the stack.
Note that the callee's rules fall cleanly into two halves that are basically mirror images of one another. The outset half of the rules apply to the kickoff of the part, and are ordinarily said to define the prologue to the function. The latter half of the rules apply to the end of the function, and are thus commonly said to define the epilogue of the function.
Example
Hither is an example office definition that follows the callee rules:
.486 .MODEL FLAT .CODE PUBLIC _myFunc _myFunc PROC ; Subroutine Prologue push ebp ; Salve the old base arrow value. mov ebp, esp ; Set the new base pointer value. sub esp, 4 ; Make room for one 4-byte local variable. button edi ; Save the values of registers that the part push esi ; will modify. This function uses EDI and ESI. ; (no demand to save EBX, EBP, or ESP) ; Subroutine Trunk mov eax, [ebp+eight] ; Move value of parameter 1 into EAX mov esi, [ebp+12] ; Move value of parameter 2 into ESI mov edi, [ebp+16] ; Movement value of parameter 3 into EDI mov [ebp-iv], edi ; Movement EDI into the local variable add [ebp-4], esi ; Add ESI into the local variable add eax, [ebp-four] ; Add the contents of the local variable ; into EAX (final result) ; Subroutine Epilogue pop esi ; Recover register values pop edi mov esp, ebp ; Deallocate local variables popular ebp ; Restore the caller'due south base of operations pointer value ret _myFunc ENDP END
The subroutine prologue performs the standard actions of saving a snapshot of the stack pointer in EBP (the base pointer), allocating local variables past decrementing the stack pointer, and saving annals values on the stack.
In the body of the subroutine nosotros can see the utilise of the base arrow. Both parameters and local variables are located at constant offsets from the base arrow for the duration of the subroutines execution. In item, we notice that since parameters were placed onto the stack earlier the subroutine was chosen, they are always located below the base pointer (i.east. at college addresses) on the stack. The first parameter to the subroutine tin always be found at memory location EBP + eight, the 2nd at EBP + 12, the third at EBP + 16. Similarly, since local variables are allocated afterwards the base of operations arrow is set, they ever reside above the base arrow (i.due east. at lower addresses) on the stack. In detail, the get-go local variable is always located at EBP - 4, the 2nd at EBP - eight, and then on. This conventional utilize of the base of operations arrow allows united states to quickly identify the employ of local variables and parameters within a part trunk.
The function epilogue is basically a mirror image of the role prologue. The caller's register values are recovered from the stack, the local variables are deallocated past resetting the stack pointer, the caller'southward base pointer value is recovered, and the ret instruction is used to return to the advisable lawmaking location in the caller.
Using these Materials
These materials are released under a Creative Commons Attribution-Noncommercial-Share Alike 3.0 United States License. Nosotros are delighted when people desire to employ or arrange the class materials we adult, and you are welcome to reuse and conform these materials for any not-commercial purposes (if you would like to use them for a commercial purpose, delight contact David Evans for more data). If yous do adapt or employ these materials, please include a credit like "Adjusted from materials adult for Academy of Virginia cs216 by David Evans. This guide was revised for cs216 by David Evans, based on materials originally created past Adam Ferrari many years agone, and since updated by Alan Batson, Mike Lack, and Anita Jones." and a link back to this folio.
Source: https://www.cs.virginia.edu/~evans/cs216/guides/x86.html
Posted by: youngwortagicenin.blogspot.com
0 Response to "What Is The Eax Register"
Post a Comment